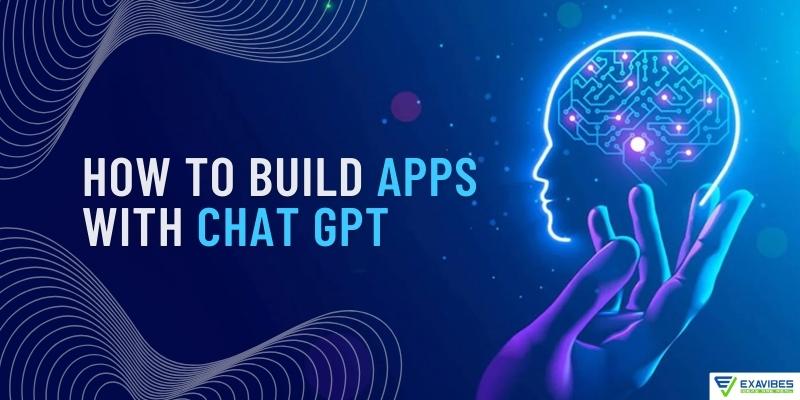
How To Build Apps With ChatGPT
What is ChatGPT?
OpenAI created ChatGPT, the largest language model chatbot. It is based on the GPT-3.5 language model and was trained on a vast text and code dataset. ChatGPT can generate text, translate languages, create other types of creative content, and answer your queries in an informed manner, even if they are open-ended, difficult, or unusual.ChatGPT is specifically fine-tuned for conversational tasks, enabling it to produce coherent and contextually relevant responses in a chat-like format. It has the remarkable ability to understand and generate text that feels like it's coming from a human.
What is ChatGPT Used For?
- Customer service: ChatGPT can be used to create chatbots that can answer customer questions and provide support. This can help businesses to save money on customer service costs and provide a better customer experience.
- Education: ChatGPT can be used to create educational tools that can help students learn new concepts and skills. For example, ChatGPT could be used to create a chatbot that can answer students' questions about a particular topic or provide practice problems.
- Entertainment: ChatGPT can be used to create creative content, such as poems, stories, and scripts. This can be used to generate new ideas for entertainment products or to create personalized experiences for users.
- Productivity: ChatGPT can be used to create tools that can help people with their work, such as email generators and code generators. This can save people time and effort, and help them to be more productive.
- Content Generation: Content creators and marketers use ChatGPT to generate blog posts, social media content, product descriptions, and more. It has the potential to save time and offer creative inspiration for writers.
- Language Translation: GPT can be used to develop language translation apps that instantly translate text between languages, making it useful for travellers and international businesses.
- Educational Tools: GPT can be integrated into e-learning platforms to provide personalized tutoring, answer student questions, and facilitate language learning.
- Healthcare: In the healthcare industry, GPT can assist with medical record documentation, answer patient inquiries, and provide general health information.
- Gaming: Game developers can incorporate GPT to create more immersive and interactive in-game NPCs (non-playable characters) that can hold conversations with players.
- Creative Writing Assistance: Authors and screenwriters can use GPT for brainstorming ideas, character development, and generating dialogue.
How Does ChatGPT Work?
ChatGPT works by using its knowledge of the world to generate text, translate languages, write different kinds of creative content, and answer your questions in an informative way.
When you ask ChatGPT a question, it uses its knowledge to search for the most relevant information in its dataset. It then uses this information to generate a response that is tailored to your specific query.
ChatGPT is still under development, but it has learned to perform many kinds of tasks, including:
- Following your instructions and completing your requests thoughtfully.
- Using its knowledge to answer your questions in a comprehensive and informative way, even if they are open ended, challenging, or strange.
- Generating different creative text formats of text content, like poems, code, scripts, musical pieces, email, letters, etc. It will try its best to fulfill all your requirements.
ChatGPT is accessible through OpenAI's API, and can be used to create a variety of applications, including chatbots, virtual assistants, and creative writing tools.
Here is a simplified overview of how ChatGPT works:
- ChatGPT receives a query from the user.
- ChatGPT uses its knowledge of the world to search for the most relevant information in its dataset.
- ChatGPT uses this information to generate a response that is tailored to the user's query.
- ChatGPT returns the response to the user.
ChatGPT is a powerful tool that has the potential to revolutionize the way we interact with computers. As ChatGPT continues to learn and improve, we can expect to see even more innovative and creative applications for this technology.
Ways ChatGPT helps in creating Apps
Understanding how ChatGPT works is crucial for building apps with this technology. Here's a simplified overview:Here are some specific ways that you can use ChatGPT to create apps:
Generate code snippets : ChatGPT can generate code snippets in a variety of programming languages, including Python, Java, and JavaScript. This can save you a lot of time and effort, especially if you are new to coding.
Debug your code : ChatGPT can help you to debug your code by identifying and fixing errors. This can save you a lot of time and frustration.
Test your app : ChatGPT can help you to test your app by simulating user interactions and generating test data. This can help you to ensure that your app is working as expected.
Generate ideas for UI/UX elements : ChatGPT can generate ideas for UI/UX elements, such as buttons, menus, and navigation bars. This can help you to create a user interface that is both user-friendly and visually appealing.
Create mockups : ChatGPT can help you to create mockups of your app's UI. This can help you to visualize your app and make changes before you start coding.
How to create Apps using ChatGPT
Here is the step by step guide on how you can create apps using ChatGPT1. Setting Up Your Development Environment
Before you can start building your ChatGPT-powered app, you need to set up your development environment. This involves the installation of the required tools and libraries.Depending on your chosen programming language and platform, the requirements may vary. Some common steps include:
Requirements:
- A development machine with internet access.
- A registered OpenAI API key for accessing ChatGPT.
- Basic knowledge of programming and API integration.
Choosing a Programming Language:
You can build GPT-powered apps in various programming languages, including Python, JavaScript, Ruby, and more. Choose the language that you're most comfortable with or that aligns with your project's requirements.
Installing Dependencies:
Install any required libraries or SDKs for your chosen programming language. For Python, you can use packages like 'requests' for API requests and libraries for NLP tasks like spaCy or NLTK.
2. Choosing the Right Use Case:
To successfully build a ChatGPT-powered app, it's crucial to identify a clear use case. What problem will your app solve, and how will users interact with it? Here are some common use cases for GPT-powered apps:Customer Support Chatbots: Provide instant assistance and support to customers on your website or platform.
Content Generation Tools: Automate content creation, such as generating product descriptions, articles, or creative writing.
Virtual Personal Assistants: Create a virtual assistant to help users with tasks like scheduling appointments or answering questions.
Language Translation Services: Build a chatbot that can translate text between languages.
Educational Assistants: Develop an AI tutor to assist students with homework or provide explanations on various topics.
Once you've chosen a use case, it's time to define the user interactions your app will support. What questions will users ask? What actions should the app be able to perform? Understanding these interactions will guide the development process.
3. Building the App
Now that you've identified your use case and defined user interactions, it's time to start building your ChatGPT-powered app. In this section, we'll guide you through the technical aspects of creating your application.Connecting to the ChatGPT API
To integrate GPT into your app, you'll need to make API requests to OpenAI's servers. Here's a simplified example in Python using the 'requests' library:
import requests
api_key = 'YOUR_API_KEY'
api_url = 'https://api.openai.com/v1/chat/completions'
def chat_with_gpt(user_message):
headers = {
'Authorization': f'Bearer {api_key}',
}
data = {
'messages': [
{'role': 'system', 'content': 'You are a helpful assistant.'},
{'role': 'user', 'content': user_message},
]
}
response = requests.post(api_url, headers=headers, json=data)
return response.json()['choices'][0]['message']['content']
# Example usage:
user_input = 'Tell me a joke.'
response = chat_with_gpt(user_input)
print(response)
This code sends a user message to the GPT API and receives a response. Please make sure to substitute 'YOUR_API_KEY' with your real OpenAI API key.
Handling User Inputs
In the code snippet above, the 'user_input' variable represents the message from the user. You can capture user input from various sources, such as a web form, chat widget, or mobile app interface, and pass it to the 'chat_with_gpt' function.
Generating Responses
The 'chat_with_gpt' function takes the user message and sends it to ChatGPT via the API. GPT generates a response, which you can extract from the API response and present to the user. You can further customize the system message and user message for context.
4. Enhancing the User Experience
Creating a seamless and engaging user experience is essential for the success of your ChatGPT-powered app. Here are some ways to enhance the user experience:Customizing ChatGPT's Responses
You can customize GPT's responses to match the tone and purpose of your application. Experiment with different system messages to guide the AI's behaviour and generate more contextually relevant responses.
Implementing Multimodal Inputs and Outputs
While ChatGPT primarily deals with text inputs and outputs, you can extend its capabilities by integrating with other AI models for image recognition, speech processing, or even incorporating rich media like videos and audio.
Handling Errors Gracefully
Consider scenarios where GPT might provide inaccurate or inappropriate responses. Implement error-handling mechanisms to gracefully handle such situations, perhaps by prompting the user to rephrase their query or providing alternative solutions.
5. Testing and Iterating
Testing is a crucial phase in the development of your ChatGPT-powered app. It ensures that your app functions as intended and provides a smooth user experience. Here's how to approach testing and iteration:Quality Assurance
- Functional Testing: Verify that ChatGPT responds correctly to user inputs and performs the desired actions.
- Usability Testing: Involve real users to assess how well your app meets their needs and expectations.
- Performance Testing: Check for bottlenecks and optimize response times, especially if your app experiences high traffic.
Gathering User Feedback
Gather input from your users to pinpoint areas that require enhancement. Consider implementing features like user ratings and feedback forms within your app. Listen to user suggestions and prioritize enhancements accordingly.
Making Iterative Improvements
Use the feedback and data collected during testing to make iterative improvements to your app. Continuously refine the AI's responses, enhance user interfaces, and address any reported issues.
6. Scaling and Deployment
As your ChatGPT-powered app matures, you'll need to prepare for scalability and deployment. Here's how to do it:Preparing for High Traffic
- Load Testing: Simulate high-traffic scenarios to identify potential performance bottlenecks and scale your infrastructure accordingly
- .Scalability Planning: Implement strategies like load balancing and auto-scaling to handle increased demand.
Deploying Your ChatGPT-Powered App
- Choose a Hosting Environment: Select a reliable hosting environment that suits your app's requirements, whether it's a cloud provider or dedicated servers.
- Deployment Pipeline: Set up a robust deployment pipeline with automated testing and continuous integration to ensure smooth updates.
Monitoring and Maintenance
- Monitoring Tools: Implement monitoring tools to track app performance, detect errors, and receive alerts when issues arise.
- Scheduled Maintenance: Plan for regular maintenance windows to apply updates, security patches, and improvements without disrupting users.
7. Legal and Ethical Considerations
As you develop your GPT-powered app, it's essential to address legal and ethical considerations:Data Privacy
- Ensure compliance with data privacy regulations like GDPR or CCPA when handling user data.
- Clearly communicate your data usage policies and obtain user consent when necessary.
Ensuring Ethical AI Usage
- Establish ethical guidelines for your app to prevent harmful or biased behaviour.
- Implement content filtering to prevent the generation of inappropriate or offensive content.
Compliance with Regulations
- Stay up-to-date with AI-related regulations and guidelines in your jurisdiction.
- Collaborate with legal experts to ensure your app complies with relevant laws.
Examples of ChatGPT Powered Apps
Here are some examples of ChatGPT-powered apps that have made a significant impact:
- ChatGPT powered chatbots: ChatGPT can be used to create chatbots that can answer customer questions and provide support. For example, Google AI is using ChatGPT to develop a new chatbot that can help people with their search queries.
- ChatGPT powered virtual assistants: ChatGPT can be used to create virtual assistants that can help people with a variety of tasks, such as managing their calendar, scheduling appointments, and ordering food. For example, Rasa is using ChatGPT to develop a chatbot that can help restaurant customers to order food and make reservations.
- ChatGPT powered creative writing tools: ChatGPT can be used to create tools that can help people with their creative writing. For example, Narrative Science is using ChatGPT to generate news articles from data.
- ChatGPT powered code generators: ChatGPT can be used to create tools that can help people generate code. For example, GitHub Copilot is a tool that uses ChatGPT to generate code suggestions for developers.
- ChatGPT powered educational tools: ChatGPT can be used to create educational tools that can help students learn new concepts and skills. For example, Duolingo is using ChatGPT to create personalized language learning exercises.
Future Possibilities and Trends in ChatGPT
The field of ChatGPT and AI in general is continuously evolving.Here are a few potential future developments and emerging trends to keep an eye on:
The future possibilities and trends of ChatGPT and similar natural language processing models are exciting and likely to have a significant impact on various industries and applications. Here are some potential future directions and trends:
-
Improved Natural Language Understanding: Future iterations of ChatGPT are likely to exhibit even better natural language understanding, allowing for more context-aware and nuanced conversations. This could lead to more accurate and context-aware responses in various applications.
-
Multimodal Capabilities: Integrating text-based models like ChatGPT with visual and auditory models to enable multimodal understanding and generation is a promising trend. This could enhance applications in content creation, accessibility, and more.
-
Domain-Specific Specialization: ChatGPT models tailored to specific industries or domains, such as healthcare, law, finance, or education, could provide highly specialized and accurate assistance in those fields.
-
Personalization: ChatGPT-powered systems that offer personalized responses and recommendations based on individual user profiles and preferences will become more prevalent. This can be applied in e-commerce, content recommendations, and virtual assistants.
-
Ethical and Bias Mitigation: There will be a continued focus on addressing ethical concerns and bias in AI models like ChatGPT. Efforts to reduce biases, ensure fairness, and promote ethical use will shape the development of these models.
-
Real-Time Collaboration: ChatGPT could be used in real-time collaboration tools, facilitating better communication and collaboration among teams, especially in remote work settings.
-
Conversational Commerce: ChatGPT will play a significant role in conversational commerce, helping customers make purchasing decisions, answering product-related questions, and providing personalized shopping experiences.
-
Education and Learning Assistants: ChatGPT-powered learning assistants will provide students with personalized tutoring, explanations, and guidance, making online education more effective.
-
Healthcare and Diagnosis: In the healthcare sector, ChatGPT models can assist with patient inquiries, provide health information, and support diagnostic processes.
-
Enhanced Content Creation: Content creators will continue to use ChatGPT to generate high-quality written content, design marketing materials, and assist in creative endeavors.
-
Emotional Intelligence: Future models may exhibit improved emotional intelligence, enabling more empathetic and emotionally aware interactions in customer support and mental health applications.
-
Multilingual and Cross-Language Support: Expect models like ChatGPT to become more proficient in multiple languages and offer seamless cross-language conversations.
-
Augmented Reality and Virtual Reality: Integration with AR and VR technologies will enable immersive and interactive conversations with virtual entities, enhancing gaming and training experiences.
-
Regulatory and Ethical Frameworks: Governments and organizations will establish clearer regulations and ethical guidelines for the use of AI models like ChatGPT to ensure responsible and ethical deployment.
-
Open-Source and Collaboration: Collaboration between researchers and organizations will continue to drive advancements in AI models. Open-source initiatives and shared research will contribute to the development of more capable models.
-
Edge Computing: Models like ChatGPT may be optimized for edge devices, allowing for more privacy-preserving and efficient AI interactions.
-
Human-AI Collaboration: The future will see increased collaboration between humans and AI, with AI assisting and augmenting human capabilities in various tasks.
Conclusion
Building apps with ChatGPT opens up exciting possibilities for developers to create conversational AI experiences that enhance user engagement and productivity.
As you embark on your journey to build ChatGPT-powered apps, remember to stay informed about the latest developments in AI and NLP. The key to success is not only technical prowess but also a commitment to delivering valuable and ethical solutions to users.
Now, armed with the knowledge from this comprehensive guide, you're well-equipped to embark on your ChatGPT app development journey. Innovate, iterate, and create remarkable conversational experiences for your users!
You can also visit related blogs:
comments for "An Interview with Exavibes Services"
Leave a Reply